Javafx Textfield Text Color
In JavaFX, a TextField is a user interface control that allows users to enter text. By default, the text color of a TextField is determined by the system or application theme. However, there are times when you may want to customize the text color of a TextField to better suit your application’s design or requirements.
Fortunately, JavaFX provides several ways to set the text color of a TextField. In this article, we will explore different techniques to change the text color in a JavaFX TextField, including using the setTextFill() method, applying CSS styles, creating custom TextFields, dynamically changing the text color based on user input, applying conditional formatting, and using external libraries for advanced text color manipulation.
Using the setTextFill() Method to Change the Text Color
The simplest way to set the text color of a TextField in JavaFX is by using the setTextFill() method. This method is available on the TextField class and allows you to specify a Color object to use as the text color. Here’s an example:
“`
TextField textField = new TextField();
textField.setText(“Hello, World!”);
textField.setTextFill(Color.RED);
“`
In the above code, we create a new TextField, set its initial text to “Hello, World!”, and then use the setTextFill() method to change the text color to red. You can specify any valid color using the Color class from the javafx.scene.paint package.
Applying CSS Styles to Modify the Text Color
JavaFX supports the use of CSS (Cascading Style Sheets) to style its controls, including TextFields. This means you can apply CSS styles to modify the text color of a TextField. To do this, you need to add a CSS selector for the TextField and specify the desired text color using the “text-fill” property. Here’s an example:
“`
TextField textField = new TextField();
textField.setText(“Hello, World!”);
textField.setStyle(“-fx-text-fill: red;”);
“`
In the above code, we create a new TextField, set its initial text to “Hello, World!”, and then use the setStyle() method to apply a CSS style that sets the text color to red. The “-fx-text-fill” property is used to specify the text color in CSS.
Creating Custom TextFields with Different Text Color
If you need to create a TextField with a specific text color that is different from the default, you can extend the TextField class and customize its appearance. Here’s an example:
“`
public class CustomTextField extends TextField {
public CustomTextField() {
super();
setStyle(“-fx-text-fill: green;”);
}
}
“`
In the above code, we create a new class called CustomTextField that extends the TextField class. In the constructor of the CustomTextField class, we set the text color to green using the setStyle() method. Now, whenever we create an instance of CustomTextField, it will have the specified text color.
Changing the Text Color Dynamically based on User Input
Sometimes, you may want to change the text color of a TextField dynamically based on user input or certain conditions. You can achieve this by adding event listeners and updating the text color accordingly. Here’s an example:
“`
TextField textField = new TextField();
textField.textProperty().addListener((observable, oldValue, newValue) -> {
if (newValue.equals(“red”)) {
textField.setTextFill(Color.RED);
} else if (newValue.equals(“blue”)) {
textField.setTextFill(Color.BLUE);
} else {
textField.setTextFill(Color.BLACK);
}
});
“`
In the above code, we add a listener to the textProperty of the TextField. Whenever the text changes, the listener checks the new value and sets the text color accordingly. If the text is “red”, it sets the text color to red. If the text is “blue”, it sets the text color to blue. Otherwise, it sets the text color to black.
Applying Conditional Formatting to TextField Text Color
Conditional formatting allows you to change the text color of a TextField based on certain conditions or rules. It can be useful for highlighting specific values or indicating errors. One way to apply conditional formatting is by using the setTextFill() method inside if-else conditions, as shown in the previous example.
Another approach is to use a binding expression that evaluates an expression and dynamically sets the text color based on the result. Here’s an example:
“`
TextField textField = new TextField();
BooleanBinding isValueValid = textField.textProperty().isEqualTo(“Valid”);
textField.textFillProperty().bind(
Bindings.when(isValueValid)
.then(Color.GREEN)
.otherwise(Color.RED)
);
“`
In the above code, we create a BooleanBinding called isValueValid that checks whether the text of the TextField is equal to “Valid”. Then, we bind the textFillProperty of the TextField to the result of the binding expression. If the text is “Valid”, the text color will be green. Otherwise, it will be red.
Using the setStyle() Method to Customize Text Color
In addition to setting the text color using the setTextFill() method, you can also use the setStyle() method to customize the text color of a TextField. This allows you to apply more complex styles or use CSS properties not available through the setTextFill() method. Here’s an example:
“`
TextField textField = new TextField();
textField.setText(“Hello, World!”);
textField.setStyle(“-fx-text-fill: linear-gradient(from 0% 0% to 100% 200%, #ff0000, #0000ff);”);
“`
In the above code, we create a new TextField, set its initial text to “Hello, World!”, and then use the setStyle() method to apply a complex text color using a linear gradient. The “-fx-text-fill” property is used to specify the text color in CSS, and the value is a linear gradient from red (#ff0000) to blue (#0000ff).
Using External Libraries for Advanced Text Color Manipulation
If you require more advanced text color manipulation in JavaFX, there are external libraries available that provide additional functionality. One such library is RichTextFX, which offers rich text editing capabilities including the ability to set text color, background color, and apply various text formatting options.
To use RichTextFX, you need to add the library as a dependency to your project. Once added, you can create a RichTextField and use its API to customize the text color. Here’s an example:
“`
import org.fxmisc.richtext.StyleClassedTextArea;
StyleClassedTextArea textArea = new StyleClassedTextArea();
textArea.append(“Hello, World!”);
textArea.setStyle(“-fx-text-fill: red;”);
“`
In the above code, we import the StyleClassedTextArea class from the RichTextFX library, create a new instance of it, append text to it, and then use the setStyle() method to set the text color to red. The StyleClassedTextArea class provides a rich set of APIs for manipulating text color and other formatting options.
FAQs
Q: How do I change the prompt text color of a JavaFX TextField?
A: To change the prompt text color of a TextField, you can use CSS styles. Set the “-fx-prompt-text-fill” property to the desired color value. Here’s an example:
“`
TextField textField = new TextField();
textField.setPromptText(“Enter your name”);
textField.setStyle(“-fx-prompt-text-fill: gray;”);
“`
Q: How do I render text in JavaFX?
A: In JavaFX, you can render text using the Text class. You can set its text, font, text color, and other properties to customize the rendered text. The Text class provides a rich set of APIs for text rendering and manipulation.
Q: How do I set the color of a label in JavaFX?
A: To set the color of a Label in JavaFX, you can use CSS styles. Set the “-fx-text-fill” property to the desired color value. Here’s an example:
“`
Label label = new Label(“Hello, World!”);
label.setStyle(“-fx-text-fill: blue;”);
“`
Q: How do I apply CSS styles to a TextField in JavaFX?
A: To apply CSS styles to a TextField in JavaFX, use the setStyle() method and provide a valid CSS style string. You can specify the desired text color using the “-fx-text-fill” property. Here’s an example:
“`
TextField textField = new TextField();
textField.setStyle(“-fx-text-fill: red;”);
“`
Q: How do I change the background color of a JavaFX TextArea?
A: To change the background color of a TextArea in JavaFX, you can use CSS styles. Set the “-fx-background-color” property to the desired color value. Here’s an example:
“`
TextArea textArea = new TextArea();
textArea.setStyle(“-fx-background-color: yellow;”);
“`
Q: How do I change the font of a JavaFX TextField?
A: To change the font of a TextField in JavaFX, you can use CSS styles. Set the “-fx-font-family” property to the desired font name, and optionally set other font-related properties such as “-fx-font-size” and “-fx-font-weight”. Here’s an example:
“`
TextField textField = new TextField();
textField.setStyle(“-fx-font-family: Arial; -fx-font-size: 16; -fx-font-weight: bold;”);
“`
Q: How do I use the setStyle() method to customize the text color of a JavaFX TextField?
A: The setStyle() method allows you to customize the text color of a TextField in JavaFX by providing a valid CSS style string. You can set the “-fx-text-fill” property to the desired color value. Here’s an example:
“`
TextField textField = new TextField();
textField.setStyle(“-fx-text-fill: green;”);
“`
Q: Are there any libraries available for advanced text color manipulation in JavaFX?
A: Yes, there are several libraries available that provide advanced text color manipulation capabilities in JavaFX. One such library is RichTextFX, which offers rich text editing features including the ability to set text color, background color, and apply various text formatting options.
Javafx Textfield ????
How To Change The Color Of Text In Textfield In Javafx?
JavaFX is a powerful framework for creating user interfaces in Java. One of its key components is the TextField, which allows users to input and edit text. By default, the text in a TextField is displayed in a black color. However, there may be instances where you need to change the color of the text to suit your application’s design or user’s preference. In this article, we will explore various approaches to change the text color in a TextField in JavaFX.
Setting the Text Color using CSS
One way to change the color of the text in a TextField is by using CSS (Cascading Style Sheets). CSS provides a flexible and robust way to control the appearance of JavaFX elements. To change the text color, we can set the -fx-text-fill property of the TextField using CSS. Here’s an example:
“`java
TextField textField = new TextField();
textField.setStyle(“-fx-text-fill: red;”);
“`
In the above example, we create a new TextField and set the -fx-text-fill property to “red” using the setStyle() method. This will make the text inside the TextField appear in red.
Changing the Text Color dynamically
In some cases, you may want to change the text color dynamically based on certain conditions or events. JavaFX provides the ability to add event listeners to JavaFX components, including the TextField. By adding an event listener, you can execute code when specific events occur, such as when the user presses a key or when the text changes. To change the text color dynamically, you can modify the -fx-text-fill property inside the event listener. Here’s an example:
“`java
textField.textProperty().addListener((observable, oldValue, newValue) -> {
if (newValue.length() > 10) {
textField.setStyle(“-fx-text-fill: green;”);
} else {
textField.setStyle(“-fx-text-fill: black;”);
}
});
“`
In the above example, we add a change listener to the textProperty of the TextField. Inside the listener, we check if the length of the new text is greater than 10. If it is, we set the -fx-text-fill property to “green”; otherwise, we set it to “black”. This will change the text color to green when the length of the text exceeds 10 characters.
Using Inline Styles
Another approach to change the text color of a TextField is by using inline styles. Inline styles are applied directly to a specific TextField instance, rather than using CSS. Inline styles provide a concise way to modify the appearance of individual JavaFX components. Here’s an example:
“`java
textField.setStyle(“-fx-text-fill: blue;”);
“`
In the above example, we set the -fx-text-fill property directly on the TextField instance to “blue” using setStyle(). This will make the text inside the TextField appear in blue.
FAQs:
Q: Can I change the text color of a TextField to a custom color using CSS?
A: Yes, you can specify any valid CSS color value for the -fx-text-fill property. For example, you can use hexadecimal color codes (#RRGGBB), RGB values, or predefined color names.
Q: How can I change the text color dynamically based on user input?
A: You can add a change listener to the textProperty of the TextField, and inside the listener, check the value of the text and modify the -fx-text-fill property accordingly.
Q: Is it possible to change the text color of a specific range of text within a TextField?
A: No, the TextField component does not provide built-in support for changing the color of specific ranges of text. If you need this functionality, you may consider using a more advanced text editing component, such as RichTextFX.
Q: Can I change the text color of a disabled TextField?
A: By default, when a TextField is disabled, the text color is usually gray. You can override this color by setting the -fx-disabled-text-fill property using CSS or inline styles.
In conclusion, changing the color of text in a TextField in JavaFX can be easily accomplished using CSS or inline styles. By leveraging these techniques, you can enhance the visual appearance of your user interface and provide a more tailored experience for your users. If you encounter any difficulties, consult the provided FAQs section for further clarification.
How To Change Font Of Text In Javafx?
JavaFX, as a powerful and versatile graphic library, provides developers with a wide range of tools to create visually appealing user interfaces. Among these features is the ability to change the font of text dynamically. Modifying the font in JavaFX is a straightforward process and can significantly enhance the visual appeal of your applications. In this article, we will explore the different ways to change the font of text in JavaFX and discuss some frequently asked questions related to this topic.
Before we dive into the details, it’s important to note that JavaFX supports TrueType and OpenType fonts. These font formats are widely used and allow for a broad selection of fonts for your application.
Method 1: Using CSS Styles
One of the most common methods to change the font of text in JavaFX is by using CSS styles. JavaFX provides a dedicated CSS file for styling, which makes it easy to define the appearance of UI elements, including text fonts.
To begin, create a new CSS file in your project’s resources folder or any desired location. Add the following code to the CSS file:
“`
.root {
-fx-font-family: “Arial”;
-fx-font-size: 14px;
}
“`
In this example, we set the font family to “Arial” and the font size to 14 pixels. You can replace these values with any other desired font family and size.
Next, in your JavaFX code, apply the CSS style to the desired text elements. Consider a Text object named “myText”:
“`java
myText.getStyleClass().add(“root”);
“`
This line of code applies the “root” CSS style defined in your CSS file to the “myText” object, changing its font according to the specified values.
Method 2: Inline Font Styling
Another way to change the font of text dynamically is by inline styling. In this approach, you can directly modify the font properties of a Text object within your JavaFX code.
To begin, create a Text object and set its initial font properties:
“`java
Text myText = new Text(“Hello, World!”);
myText.setFont(Font.font(“Arial”, FontWeight.BOLD, 18));
“`
In the example above, we set the font family to “Arial”, the font weight to “BOLD”, and the font size to 18. You can adjust these values as per your requirements.
To change the font dynamically, you can use the setFont() method and provide new font values:
“`java
myText.setFont(Font.font(“Times New Roman”, FontWeight.NORMAL, 16));
“`
Here, we change the font family to “Times New Roman”, the font weight to “NORMAL”, and the font size to 16. Once again, these values can be customized based on your design preferences.
FAQs:
Q: Can I use custom fonts in JavaFX?
A: Yes, JavaFX supports custom fonts. To use a custom font, you need to load the font file using the Font.loadFont() method and then set it as the font for your text element.
Q: How can I change the font color along with the font family?
A: In both CSS and inline styling, you can modify the font color using the -fx-fill property. For example, to set the font color to red in CSS:
“`
.root {
-fx-font-family: “Arial”;
-fx-font-size: 14px;
-fx-fill: red;
}
“`
Q: Can I change the font of other UI elements, such as labels or buttons?
A: Yes, you can change the font of various UI elements in JavaFX. The process is similar to changing the font of text. Simply apply the font properties to the desired UI element using CSS or inline styling.
Q: Where can I find a list of available system fonts in JavaFX?
A: You can retrieve a list of available system fonts using the Font.getFamilies() method. This method returns an array of strings representing the available font families on the system.
In conclusion, changing the font of text in JavaFX is a simple yet powerful feature that can greatly enhance the visual appeal of your applications. Whether you prefer using CSS styles or inline styling, JavaFX provides the flexibility to customize fonts according to your design requirements. Moreover, the option to use custom fonts empowers developers to create unique and visually engaging user interfaces.
Keywords searched by users: javafx textfield text color Javafx prompt text color, Render text JavaFX, Set color label javafx, CSS textfield JavaFX, JavaFX TextArea background color, Javafx TextField, Font javafx, setStyle javafx
Categories: Top 98 Javafx Textfield Text Color
See more here: dongtienvietnam.com
Javafx Prompt Text Color
JavaFX is a powerful framework for building rich desktop applications. With its extensive set of features and components, it facilitates the development process and enables developers to create dynamic and engaging user interfaces. One such feature in JavaFX is the ability to set the prompt text color, which provides users with valuable cues and enhances the overall user experience. In this article, we will delve into the details of JavaFX prompt text color, discussing its significance, implementation, and common FAQs.
Understanding the Significance of Prompt Text Color
Prompt text color is crucial for guiding users and providing them with helpful hints or instructions. It is commonly used in input fields to display placeholder text, indicating the expected format or data that should be entered. By setting an appropriate prompt text color, developers can make the user interface more intuitive and user-friendly, leading to improved usability and overall satisfaction.
Implementing Prompt Text Color in JavaFX
JavaFX provides a convenient way to set the prompt text color for various components, such as text fields and text areas. The javafx.scene.control.TextInputControl class, which is inherited by these components, exposes the promptTextFill property. This property allows developers to define the color of the prompt text using the Color class from the javafx.scene.paint package.
To set the prompt text color, developers can follow these simple steps:
1. Retrieve the TextInputControl instance (e.g., TextField or TextArea) from the JavaFX scene graph.
2. Instantiate the Color class with the desired RGB values or use one of the predefined color constants.
3. Assign the Color instance to the promptTextFill property of the TextInputControl.
Here is an example illustrating the implementation of prompt text color in JavaFX:
“`java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.TextField;
import javafx.scene.layout.VBox;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class PromptTextColorExample extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
TextField textField = new TextField();
textField.setPromptText(“Enter your name”);
textField.setStyle(“-fx-prompt-text-fill: red;”);
VBox root = new VBox(textField);
Scene scene = new Scene(root, 300, 200);
primaryStage.setScene(scene);
primaryStage.show();
}
}
“`
In the above example, we create a TextField instance and set its prompt text to “Enter your name.” We then use the setStyle method to assign the desired prompt text color. In this case, the “-fx-prompt-text-fill: red;” style rule sets the prompt text color to red. By modifying the value of “-fx-prompt-text-fill,” developers can customize the prompt text color according to their requirements.
JavaFX Prompt Text Color FAQs:
Q1. Can we set the prompt text color for other JavaFX components, such as labels or buttons?
A1. No, the prompt text color feature is specific to Input Control components, such as TextField and TextArea, in JavaFX. For other components like labels or buttons, the prompt text color functionality is not provided by default.
Q2. Can we use CSS stylesheets to set the prompt text color?
A2. Yes, JavaFX supports the use of CSS stylesheets. Developers can define stylesheets and apply them to the desired components, including setting the prompt text color, by using CSS selectors and properties.
Q3. Is it possible to change the prompt text color dynamically at runtime?
A3. Yes, developers can modify the prompt text color dynamically during runtime by updating the promptTextFill property of the corresponding TextInputControl instance.
Q4. How can we reset the prompt text color back to its default value?
A4. To reset the prompt text color back to its default value, developers can simply set the promptTextFill property to null or do not set the promptTextFill property at all. This way, JavaFX will apply the default prompt text color defined by the current style.
In conclusion, JavaFX prompt text color plays a significant role in enhancing the user experience and guiding users through various input fields. By following the implementation guidelines provided in this article, developers can easily configure the prompt text color for Input Control components in their JavaFX applications. By leveraging this functionality effectively, developers can create intuitive and visually appealing user interfaces, resulting in an excellent user experience.
Render Text Javafx
JavaFX is a powerful and versatile framework for creating rich and interactive user interfaces. One of the essential components of any user interface is the ability to render text effectively. JavaFX provides a comprehensive set of features and tools for rendering text, making it both simple and convenient for developers to create visually appealing and user-friendly applications. In this article, we will explore the various options available in JavaFX for rendering text and provide insights into best practices and frequently asked questions.
Rendering Text in JavaFX
JavaFX offers multiple ways to render text. The primary component used for rendering text is the Text class, which allows developers to create and manipulate text objects with ease. Let’s dive into some of the essential features and techniques for rendering text in JavaFX.
1. Basic Text Rendering:
The simplest way to render text in JavaFX is by using the Text class. Here’s an example:
“`java
Text text = new Text(“Hello, JavaFX!”);
“`
This creates a Text object with the specified text content, which can be manipulated and styled as needed.
2. Styling Text:
JavaFX provides extensive styling options for text rendering. Developers can modify the font, size, color, and other properties of text effortlessly. Here’s an example of how to style text:
“`java
text.setFont(Font.font(“Arial”, FontWeight.BOLD, 20));
text.setFill(Color.RED);
“`
In this example, we set the font to Arial with bold weight and size 20. We also set the text color to red.
3. Text Wrapping and Clipping:
JavaFX allows developers to control text wrapping and clipping, ensuring that the text fits within the desired boundaries. The TextFlow class provides a container for text that automatically wraps the text content within its bounds. Additionally, developers can use the Clip class to restrict the visible portion of the text.
4. Text Alignment:
JavaFX provides flexible options for aligning text horizontally and vertically. The TextAlignment enum offers various alignment options such as LEFT, CENTER, and RIGHT for horizontal alignment, and TOP, CENTER, and BOTTOM for vertical alignment.
5. Text Effects:
JavaFX offers a range of text effects to enhance the visual appeal of rendered text. Developers can apply effects such as shadows, glows, and reflections using the Effect class. For instance:
“`java
DropShadow dropShadow = new DropShadow();
text.setEffect(dropShadow);
“`
This applies a drop shadow effect to the text.
6. Rich Text Formatting:
JavaFX supports rich text formatting through the TextFlow and Text classes. Using these classes, developers can create complex layouts with different text styles within a single text object. For example:
“`java
TextFlow textFlow = new TextFlow();
Text hello = new Text(“Hello”);
hello.setFill(Color.BLUE);
Text comma = new Text(“, “);
Text javafx = new Text(“JavaFX”);
javafx.setFill(Color.GREEN);
textFlow.getChildren().addAll(hello, comma, javafx);
“`
In this example, we create a TextFlow that contains three Text objects with different styles. The TextFlow seamlessly combines these text objects into a single layout.
Frequently Asked Questions:
Q1. Can I render text in multiple languages using JavaFX?
A1. Yes, JavaFX fully supports internationalization and rendering of text in different languages, allowing developers to create applications that cater to a global audience.
Q2. How can I apply custom fonts to rendered text?
A2. JavaFX supports loading and using custom fonts. Developers can load fonts from various sources and apply them to their text objects using the Font class.
Q3. Can I animate text in JavaFX?
A3. Yes, JavaFX provides powerful animation capabilities that can be applied to text objects. Developers can create dynamic and interactive animations for text by utilizing the animation API.
Q4. Is it possible to interact with rendered text?
A4. Yes, JavaFX allows text objects to be interactive. Developers can assign event handlers to text objects, enabling user interactions such as clicks and hover effects.
Q5. Can I render text with complex formatting, such as bullet points or subscript?
A5. While JavaFX does not provide built-in support for complex formatting like bullet points or subscript, developers can achieve these effects through custom implementations using rich text formatting techniques.
In conclusion, JavaFX offers a robust set of tools and features for rendering text in an intuitive and visually appealing manner. By leveraging the Text class along with various styling, formatting, and effect options, developers can create highly customized and interactive applications. With JavaFX’s continuous development and widespread community support, the possibilities for rendering text are endless.
Set Color Label Javafx
JavaFX is a powerful framework for building graphical user interfaces (GUIs) in Java. It provides a wide range of tools and features that allow developers to create visually appealing and interactive applications. One important aspect of GUI design is the ability to set color labels, which can enhance the user experience and provide clear visual cues. In this article, we will explore the process of setting color labels in JavaFX, including different approaches and techniques.
Setting color labels in JavaFX can be achieved using various methods. In JavaFX, the Label class provides a straightforward way to create and customize labels. To set the color of a label, we can use the setStyle() method and apply CSS styling.
Example 1: Setting a Color Label using CSS
Label label = new Label(“Hello World!”);
label.setStyle(“-fx-text-fill: red;”);
In this example, we create a new label with the text “Hello World!”. Then, we use the setStyle() method to set the CSS styling for the label. By applying the “-fx-text-fill” property, we set the text color to red.
Besides setting the text color, we can also change the background color of a label using CSS.
Example 2: Setting a Color Label with Background
Label label = new Label(“Hello World!”);
label.setStyle(“-fx-text-fill: white; -fx-background-color: blue;”);
Here, we modify the previous example by adding “-fx-background-color” to set the background color of the label. By using the RGB, HSB, or named color values, we can create labels with different color combinations.
Another approach to set color labels in JavaFX is by using the setGraphic() method. This method allows us to add any JavaFX node, including shapes, images, or other labels, as the graphical representation of a label.
Example 3: Setting a Color Label with a Shape
Rectangle rectangle = new Rectangle(100, 40);
rectangle.setFill(Color.GREEN);
Label label = new Label(“Shape Label”);
label.setGraphic(rectangle);
In this example, we create a rectangle shape with a green fill color using the Rectangle class. Then, we set the graphic of a label to be our rectangle shape. This technique can be particularly useful when we want to use custom shapes or images as labels.
Frequently Asked Questions (FAQs)
1. Can I set different text colors for different parts of a label?
Unfortunately, the Label class in JavaFX does not provide a built-in way to set different text colors for different parts of the label. However, you can achieve this by using rich text controls, such as TextFlow or WebView, which offer more advanced text formatting options.
2. How can I set the color label for a specific event or condition?
One option is to use event handlers in JavaFX, such as onMouseEntered and onMouseExited events, to change the color label dynamically based on user interactions. Alternatively, you can use bindings to associate the color label with specific properties or variables in your code, allowing it to update automatically when those values change.
3. Can I use CSS files to set color labels in JavaFX?
Yes, you can define CSS styles in external files and apply them to your JavaFX application using the setStylesheet() method. This makes it easier to manage and reuse styles across multiple labels or even different applications.
4. Are there any limitations or performance considerations when setting color labels in JavaFX?
While JavaFX provides flexibility in setting color labels, it’s important to keep performance in mind. Applying complex styles, such as gradients or blending effects, can impact the rendering performance, especially in applications with many labels or animations. Consider optimizing your code or using CSS optimizations to ensure smooth performance.
In conclusion, setting color labels in JavaFX allows developers to enhance the visual appeal and user experience of their applications. Whether through CSS styling or using graphic elements, JavaFX provides several methods to create eye-catching and informative color labels. By experimenting and combining different techniques, developers can create unique and visually appealing interfaces that improve user engagement and understanding.
Images related to the topic javafx textfield text color

Found 49 images related to javafx textfield text color theme


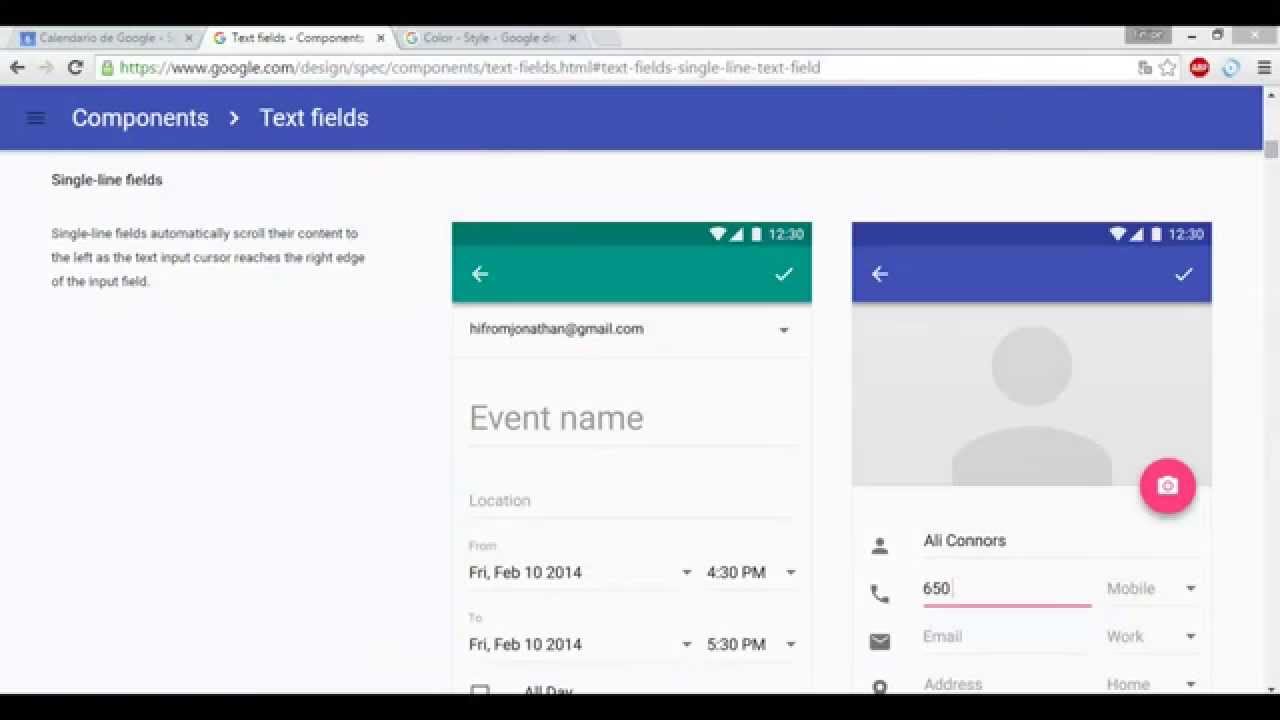
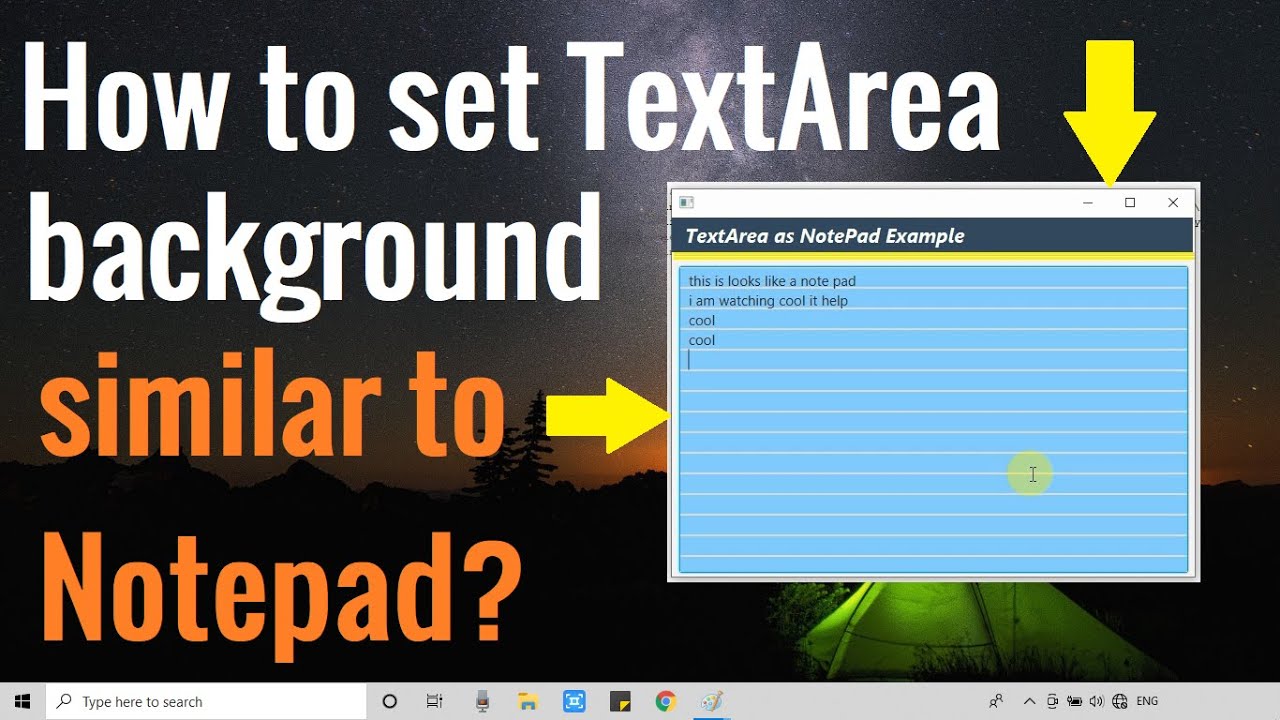
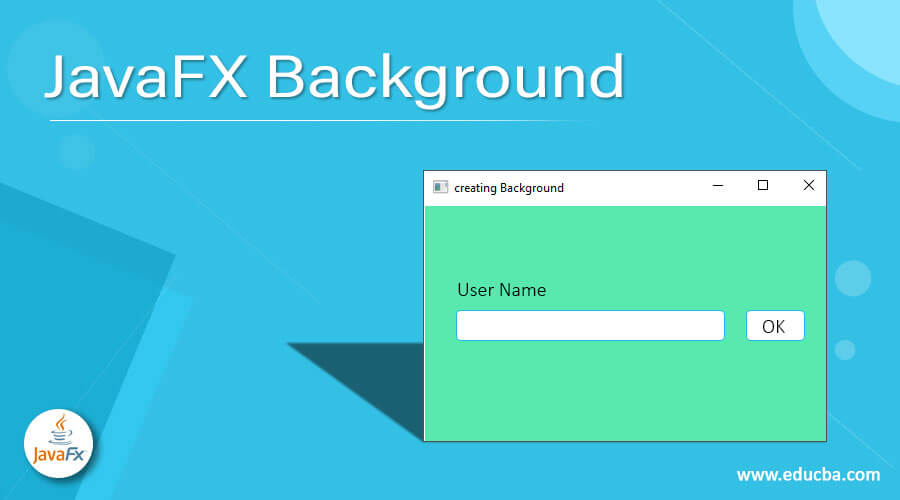
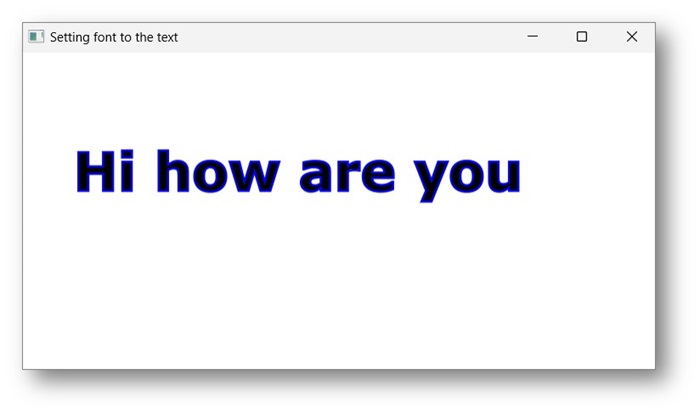
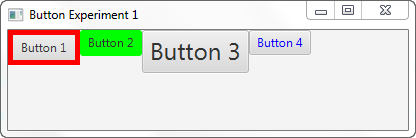
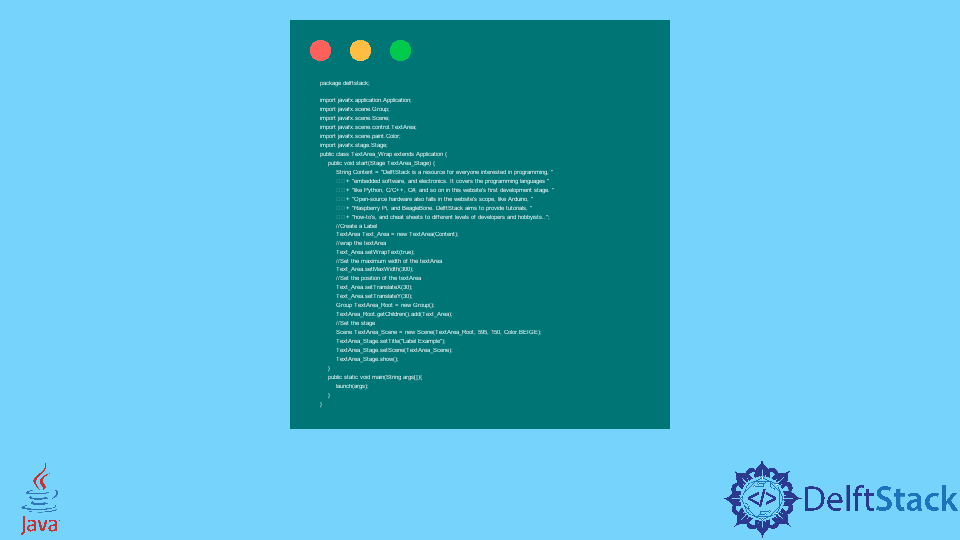
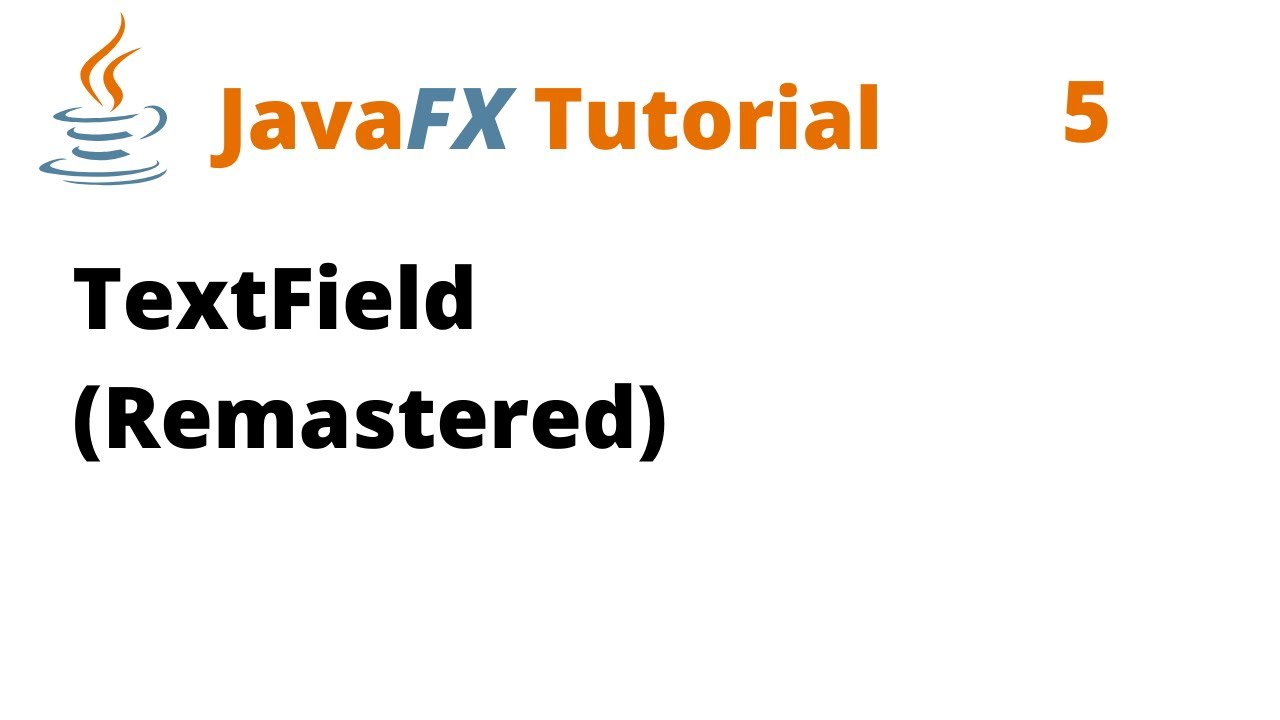
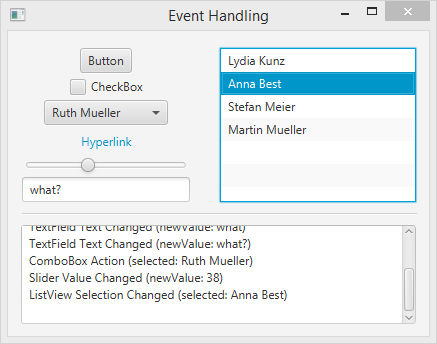
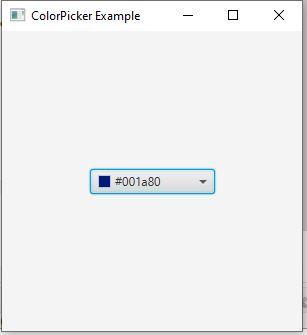

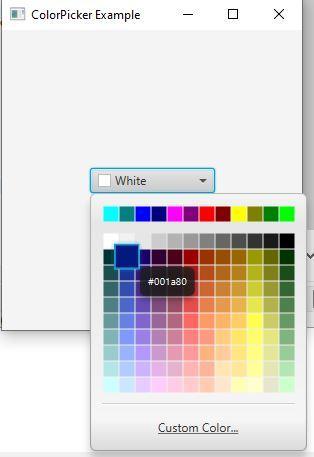

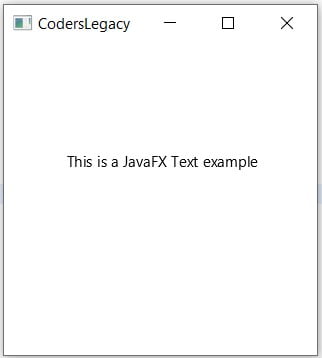
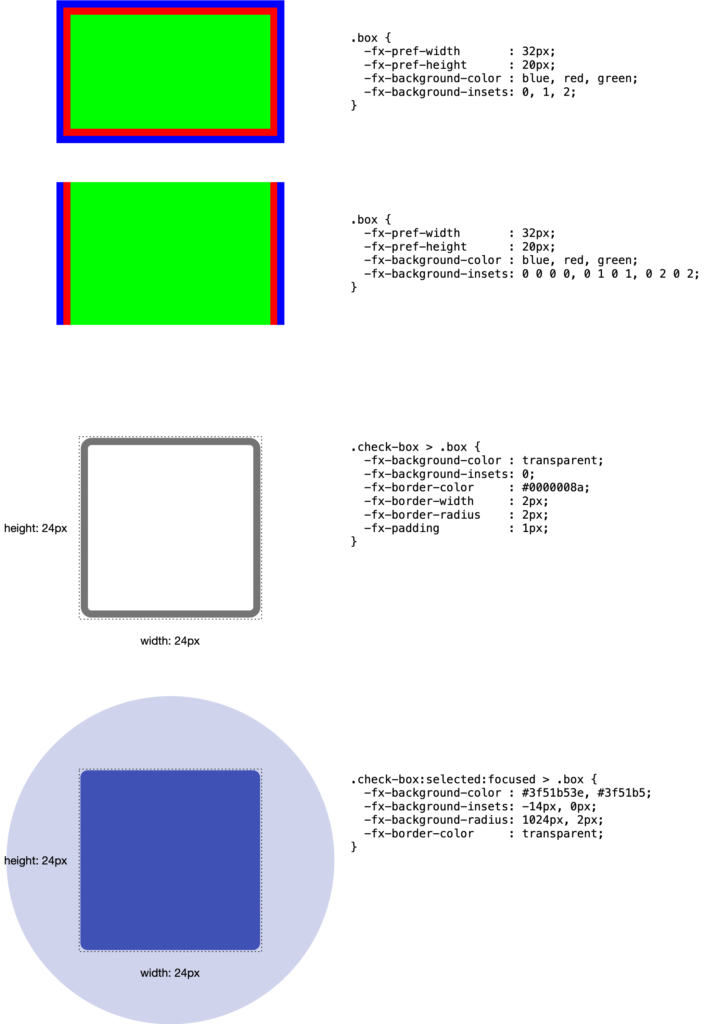
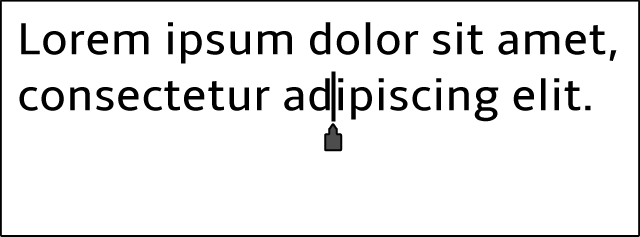
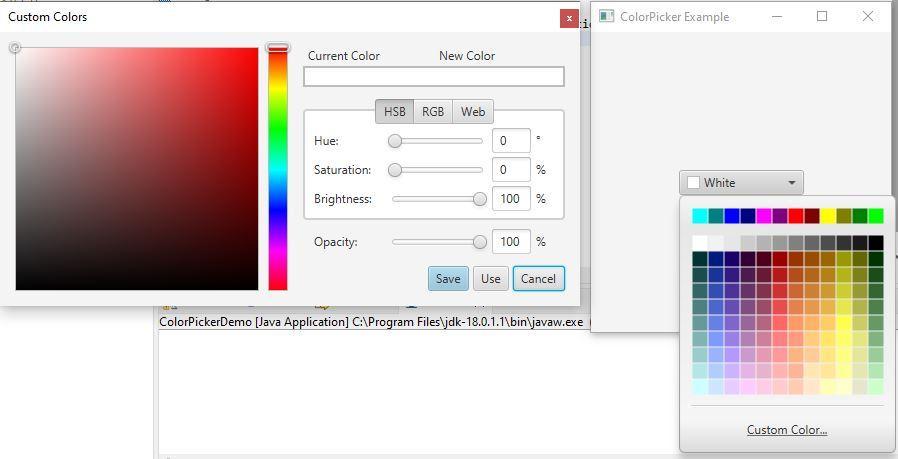
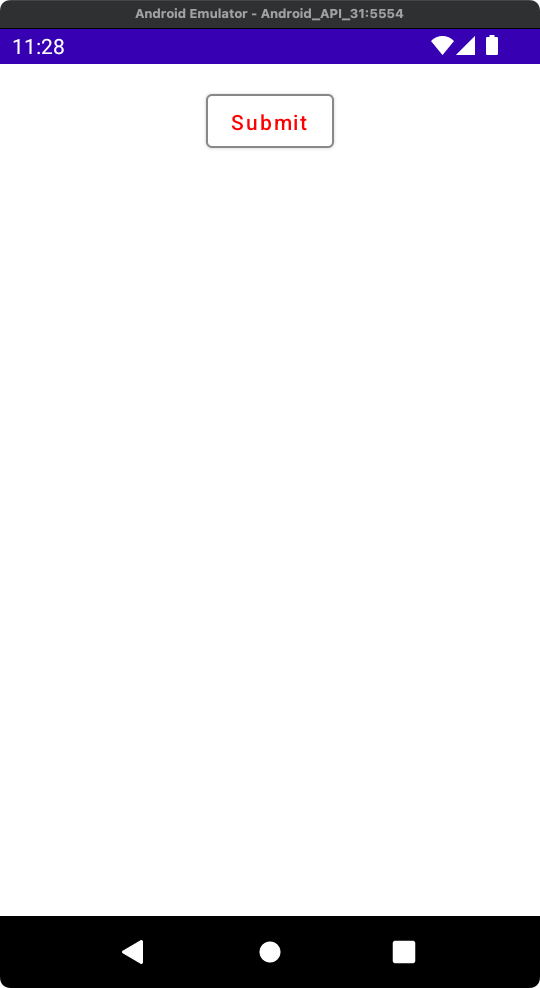
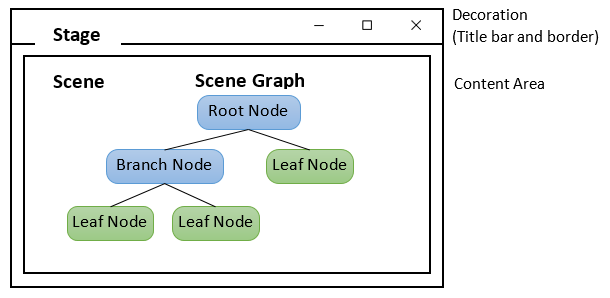
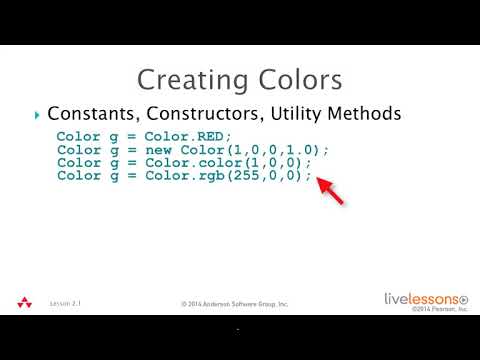
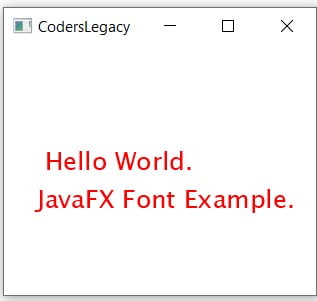
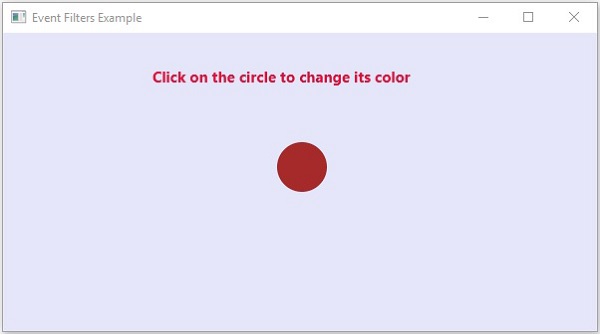
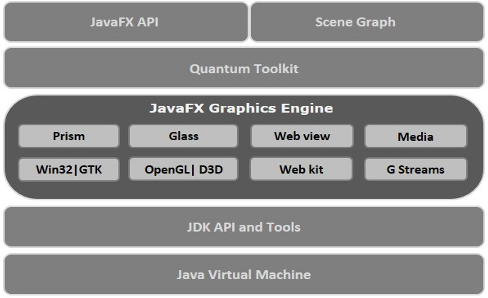
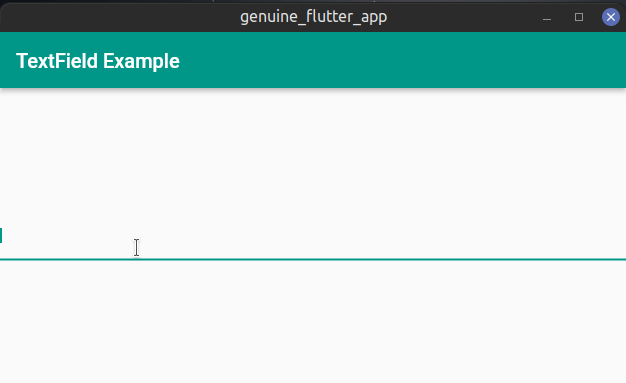

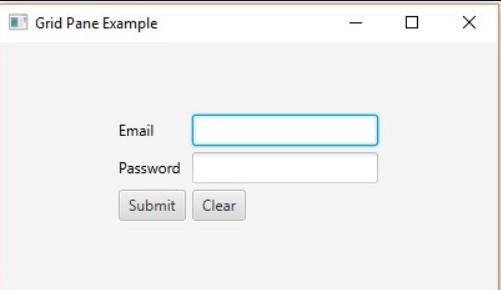

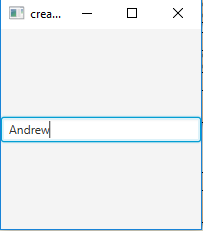

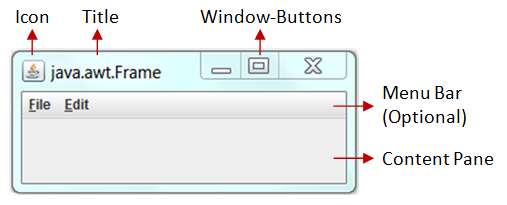
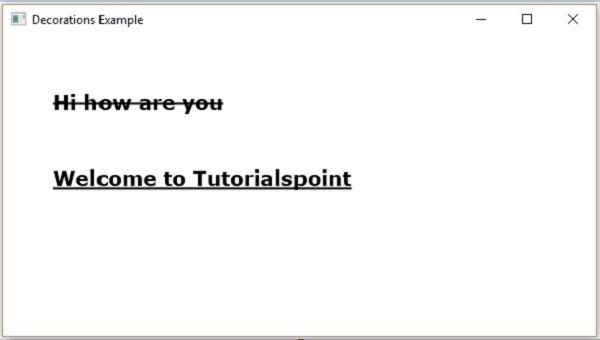
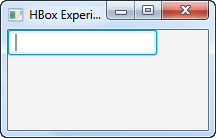
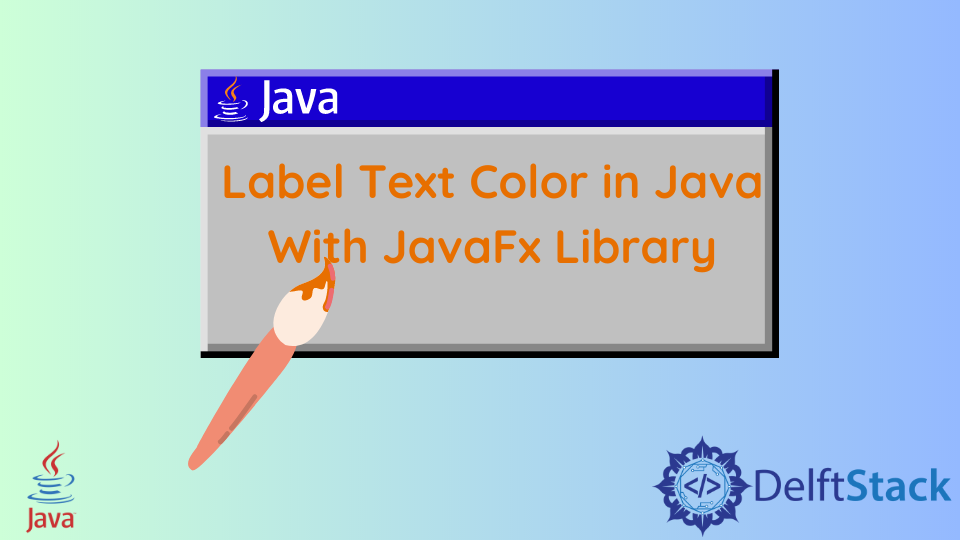
Article link: javafx textfield text color.
Learn more about the topic javafx textfield text color.
- How to change the color of text in javafx TextField?
- Color Textfield Text in JavaFX | Delft Stack
- How To Change The Color Of TextField in JavaFX?
- Using Text and Text Effects in JavaFX
- How to change the color of text in javafx TextField?
- JavaFX Text – Jenkov.com
- How To Set Border Color Of TextField in JavaFX?
- Getting Started with JavaFX: Using FXML to Create a User Interface
- How to change the color of a text box in JavaFX – Quora
- JavaFX – Text – Tutorialspoint
- How to change the color of text in javafx TextField – iTecNote
- java.awt.TextArea.setStyle java code examples – Tabnine
- How to change text color in JFXTextField? · Issue #549 – GitHub
- JavaFX Text, Font and Color Example Tutorial – Java Guides